All problems were created and prepared by me and magnus.hegdahl. Thanks to BucketPotato, Monogon, and Priyam2k for valuable feedback and testing. Special thanks to MikeMirzayanov for coming up with the idea and preparing the unique checker for 1663H - Cross-Language Program. I hope you enjoyed the contest, and found it to be an interesting series of puzzles.
Example solution code written by Agnimandur is provided for each problem.
1663A - Who Tested?
The problem statement is the title of the problem.
Re-read the original announcement blog.
If you carefully read the announcement blog, you will notice that I especially thanked BucketPotato for testing. Just print "BucketPotato".
print("bucketpotato")
1663B - Mike's Sequence
Did you notice the peculiar constraints on the variable $$$r$$$?
"Mike" is of course MikeMirzayanov. "Mike's sequence" has to do with Codeforces ratings.
3000 is the cutoff for the highest rating: Legendary Grandmaster.
Mike's sequence are the Codeforces rating thresholds: [1200,1400,1600,1900,2100,2300,2400,2600,3000]. Your task is simply to print the threshold immediately above the provided rating $$$r$$$.
R = int(input())
ratings = [1200,1400,1600,1900,2100,2300,2400,2600,3000]
higher = [r for r in ratings if r > R]
print(higher[0])
1663C - Pōja Verdon
Aenar Targaryen was a dragonlord of Old Valyria.
The main clue is the title: "Pōja Verdon".
Aenar Targaryen was a dragonlord of Valyria (an empire from a A Song of Ice and Fire) who moved his family to Westeros right before Valyria was destroyed.
pōja
means their
and verdon
means sum
(or amount
based on context) in High Valyrian (you can easily translate this on Duolingo).
In a nutshell: Print the sum of the array.
n = int(input())
nums = list(map(int,input().strip().split()))
print(sum(nums))
1663D - Is it rated - 3
Did you notice that the problem had a special "constraints" section? That's highly unusual for a Codeforces problem.
The length of S is 3. Are there any famous three letter programming contests?
The capital variable letters and the "constraints" section are designed to mimic how atcoder.jp formats their problem statements.
The problem is asking whether the Atcoder contest (ABC,ARC,AGC) is rated for a rating $$$X$$$. (Note that the judge ignores Atcoder heuristic contests for simplicity).
- ABC's are rated for $$$[0,1999]$$$
- ARC's are rated for $$$[0,2799]$$$
- AGC's are rated for $$$[1200,\infty]$$$
s = input().strip()
x = int(input().strip())
if (s=="ABC" and x<2000) or (s=="ARC" and x<2800) or (s=="AGC" and x>=1200):
print('yes')
else:
print('no')
1663E - Are You Safe?
Do you recognize the story of the Minotaur?
The problem is a word search, but not for the word "minotaur".
Perhaps you recognize the story of the minotaur? Less known is the hero who saved the kids from the minotaur: his name was Theseus [thee-see-us].
The problem is just a word search (horizontal, vertical, diagonal) for theseus
. You should only go in the forward direction when you search for a word.
The grid contains other clues embedded in it. Perhaps you can find esoteric
, java
, and script
(hints for problem G). You might be able to find atcoder
(hint for problem D). Also hero
(hinting at Theseus for this problem).
There are also some red herring words out there.
#include <bits/stdc++.h>
using namespace std;
void good() {
cout << "YES";
exit(0);
}
int main() {
int N; cin >> N;
char grid[N][N];
for (int i = 0; i < N; i++) {
string row; cin >> row;
for (int j = 0; j < N; j++) grid[i][j] = row[j];
}
string word = "theseus";
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (i < N-7) {
string w = "";
for (int k = 0; k < 7; k++) w += grid[i+k][j];
if (w == word) good();
}
if (j < N-7) {
string w = "";
for (int k = 0; k < 7; k++) w += grid[i][j+k];
if (w == word) good();
}
if (i < N-7 && j < N-7) {
string w = "";
for (int k = 0; k < 7; k++) w += grid[i+k][j+k];
if (w == word) good();
}
}
}
cout << "NO";
}
1663F - In Every Generation...
Where is the quote from?
"buffy", "the", "vampire", "slayer"
"buffy" (length 5), "the" (length 3), "vampire" (length 7), "slayer" (length 6). Remember how $$$3 \le |s| \le 7$$$? Now go back to the sample and see what you can do.
The quote is from the well known TV series Buffy the Vampire Slayer.
Perhaps you notice the lengths of the words buffy
, the
, vampire
, slayer
are unique integers in the set {3,5,6,7}.
Given the string $$$s$$$, simply "add" that string with the target word of the same length.
Here's how adding two letters work:
- Convert both letters to numbers in $$$[0,25]$$$.
- Add the two numbers up modulo 26.
- Convert the number back into a letter.
You can verify your addition algorithm is correct by checking whether "tourist" + "vampire" = "ooggqjx" (as provided in the sample).
Finally, if the length of $$$s$$$ is $$$4$$$, the answer is "none", because there is no word of length 4 in Buffy the Vampire Slayer.
s = input().strip()
words = ["","","","the","","buffy","slayer","vampire"]
if len(s)==4:
print("none")
else:
target = words[len(s)]
ans = ""
for i in range(len(s)):
a = ord(s[i])-ord('a')
b = ord(target[i])-ord('a')
ans += chr((a+b)%26 + ord('a'))
print(ans)
1663G - Six Characters
Input is a normal string of letters, but output consists of non-letter characters?
Think of an esoteric programming language.
JSF (an esoteric programming language equivalent to Javascript that only uses 6 characters).
The April Fool's Contests love of esoteric programming languages, combined with the reference to "six characters", will eventually lead you to JSFuck.
The six characters is a pun: the input is a string of length 6 like "abcdef", but the output is a string \textit{consisting} of only 6 characters: [ ] ( ) ! +
.
Brief research will demonstrate a mapping from each english letter to a JSFuck string. Two letters can be concatenated with "+".Note that the optimal mapping will make your solution file approximately 12KB in size, well within the 64KB requirement of Codeforces.
However, the mapping from each letter to javascript is not unique! However, the legend mentions that Aenar needs to go to the string's "home". JSFuck's home website is here, which is where the jury's mapping is created from.
Alternatively, instead of hardcoding the generated strings, you can simply use the encoding algorithm provided in the Github.
1663H - Cross-Language Program
Google research into how Pascal and C++ work will eventually lead you to a solution like this. Many people used the fact that \
can be used to continue single line comments to the next line in C++ but not in Pascal.
// \
begin end.
int main() {}
I did all possible word searches (normal, normal with diagonal, normal with diagonal with reverse, straight up grid BFS) for "MINOTAUR" and then for both "MINOTAUR" and "THESEUS" but didn't consider doing for just "THESEUS", fml
I did all possible word searches for
theseus
, got WA. Then I did only the forward-diagonal (i.e.[i0+k][j0+k]
) search and got AC. Meanwhile the editorial says you had to do horizontal and vertical searches too. Who's in the right?Test cases were a bit weak, you had to do horizontal, vertical, and diagonal.
IMO it is quite mean, that testcases had backwards-going "theseus" in them but were not considered safe. :D I checked for theseus first (but was also checking theseus backwards ord the other diagonal) but it only passed test 1. Then I checked for minotaur && !theseus as the unsafe condition and got to case 5.
same, smh
I printed "red panda" for problem A and got accepted???
Ha ha that's an easter egg :)
Problem A accepts either "bucketpotato" or "red panda" (or various similar combinations).
Is it normal that i wrote program writing "Red panda" for A?
what is meant by "AGC" in problem D?
See https://atcoder.jp/contests/
AGC -> AtCoder Grand Contest
Ah yes I totally forgot thanks!
is it rated?
why did my rating change ._.
Bro it is rated! No way lol
What the actual f-k?
https://codeforces.net/contest/1663/submission/152390392
This gets AC in E.
At first I thought that the generator used the same rng, but surely it doesn't work like this, right?
Maybe there are like only ~6 tests so it's not too hard for random to pass.
E has 9 tests, so in theory there's a 1/512 chance of this happening lol.
Rejudge and see how lucky they really are lmao
Actually, if I recall correctly, CF re-runs your submission two more times in case of getting wrong answer. So the probability of failing all three attempts is $$$\frac{1}{8}$$$, probability of guessing answer in one test case is $$$\frac{7}{8}$$$, while you get AC with $$$\left(\frac{7}{8}\right)^9 \approx 0.3$$$ probability. Really worth a try!
UPD: It seems that I confused WA and TLE solutions. While it is rather a well-known fact that CF re-runs TLE solutions, it is very strange to do it also with WA ones. Does CF really do it? Is it right to behave so?
CF doesn't seem to do it, because if codeforces actually did it and the chance of bypassing all 9 test with a random solution was 0.3, then with 10 tries i should get AC with 97.8% probability, but i don't. That only can mean that CF make only one attempt on failure or that i'm very unlucky :)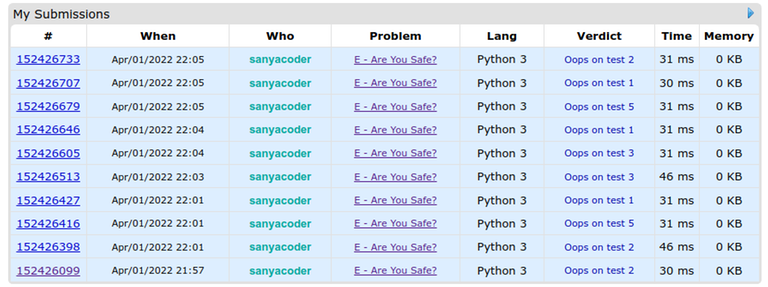
You should try playing poker.
You have chance to pass any problem if you are lucky. Just pring random strings.
can anyone tell me why I am getting WA in E? I did just like in editorial :(. My submission
wtf bro
The problem was that you accessed out-of-bounds memory; I have corrected your solution and it now gets AC: 152390393.
It is RATED! WTF???
I bet the rating changes will be reverted tomorrow :)
It's obvious, but still very unexpected
problem G
Hard-coding strings
solution code isn't availableI guess the rating changes are only for today .
Are the rating updates an April Fool's joke?
Submission Any particular reason why this code for C wasn't accepted?
Because you wrote ans=+x Instead of ans+=x
T_T
Did it work?
yes T_T
WOW
Well, it is rated :D
I write my user name in A get wrong Why
The answer is either "BucketPotato" or "Red Panda"
During the contest, after studying Pascal for an hour, I found a clumsy solution for H.
I wonder what I was doing :<
How can I become a expert? It is so hard!!!!!!!!!! T_T
Now you are a expert lol